Focus Adjustment (C++)
Focussing an event-based camera can not be done in front of a static scene like for a traditional frame-based camera. It requires to generate enough events so that a known/recognizable pattern or object can be displayed and allow for the operator to gradually adjust the optic settings until a sharp image is obtained.
Note
Metavision Studio and Metavision Viewer can show frames produced by a camera and hence can be used to focus a camera. Waving your hand in front of the camera while tuning the optic should allow you to obtain an acceptable focus. The tool we propose here goes a step further by computing a “sharpness score”.
The focusing tool of our SDK is metavision_blinking_pattern_focus
.
It uses the Calibration C++ API
to allow you to easily adjust the focus of an event-based camera.
To get the best results, the camera should be placed in front of a blinking pattern at the desired distance (that is, the distance that we want to be in focus). The blinking pattern can be generated by the tool or by other means.
The tool creates a frame from the events generated by the blinking pattern. It then computes the Discrete Fourier Transform (DFT) of the created frame and a score measuring the sharpness of the observed edges. The score determines the quality of focus: the higher the score, the sharper the edges, the better the focus.
To adjust the focus, change the aperture (if available) and focus distance (if available) on the objective lens until you get the highest score possible.
Note that the score depends on the focus but also on other factors (lens, distance from the pattern, etc), so it is not always possible to compare scores between different focusing sessions.
The source code of this tool can be found in <install-prefix>/share/metavision/sdk/calibration/cpp_samples/metavision_blinking_pattern_focus
when installing Metavision SDK from installer or packages. For other deployment methods, check the page
Path of Samples.
For an overview on how to focus an event-based camera, you can also watch our training video:
Expected Output
Metavision Focus Adjustment tool visualizes all events generated by the camera (on the left) and blinking events (on the right) with the output score indicating the sharpness of edges and thus the quality of focus:
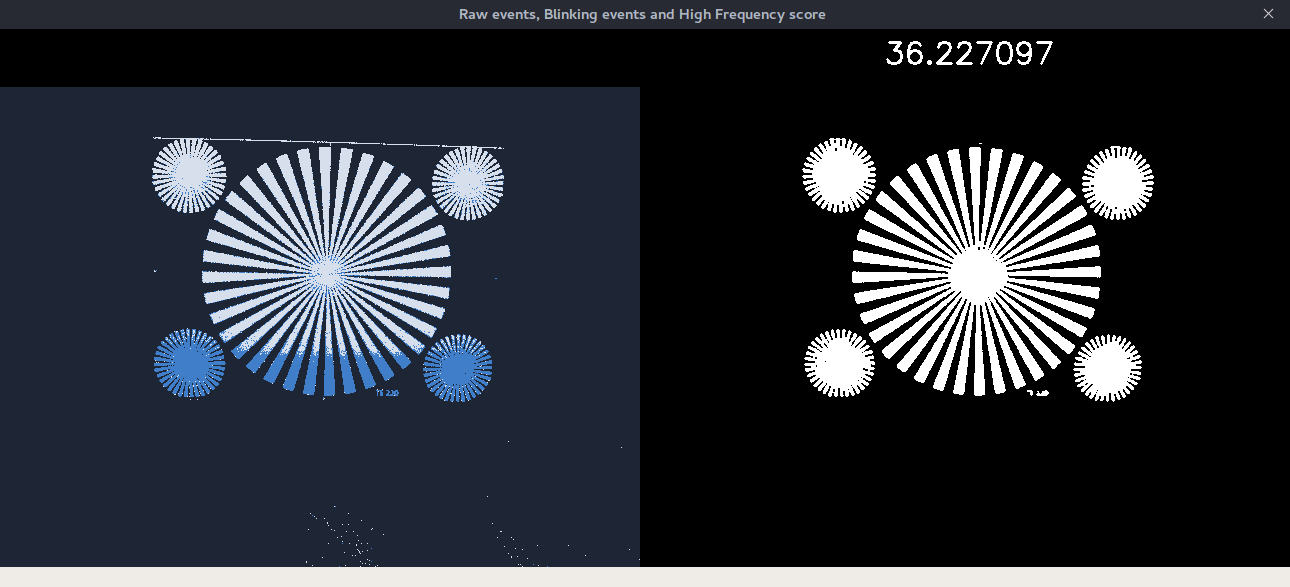
Warning
If you are using an EVK4 and are unable to obtain a good focus, make sure that you inserted the provided CS-C adapter between the camera case and the optic. Check the EVK4 unboxing video or user manual for more information.
How to start
You can either use the pre-compiled executable or compile the source code as described in this tutorial.
For the blinking pattern, you can use the pattern file blink-pattern.jpg
located in
<install-prefix>/share/metavision/sdk/calibration/cpp_samples/metavision_blinking_pattern_focus
on Ubuntu and
<install-prefix>\share\metavision\sdk\calibration\cpp_samples\metavision_blinking_pattern_focus
on Windows.
Warning
After displaying the blinking pattern, you might observe a persistent image (“after image”) on your screen. This should not be a permanent damage to your screen and should go away after a while. Nevertheless, we advise to use the flickering image only during the focusing procedure and close it when you are done.
The easiest way to use this tool is to provide a path to the pattern file on the command line. This way the pattern will be shown by the tool and you can point the camera to it:
Linux
metavision_blinking_pattern_focus --pattern-image-path PATTERN_FILE_PATH
Windows
metavision_blinking_pattern_focus.exe --pattern-image-path PATTERN_FILE_PATH
Alternatively, you can open the blinking pattern in a third-party tool, then you can start the pre-compiled executable with this command:
Linux
metavision_blinking_pattern_focus
Windows
metavision_blinking_pattern_focus.exe
To check for additional options:
Linux
metavision_blinking_pattern_focus -h
Windows
metavision_blinking_pattern_focus.exe -h
Code Overview
Optional: Blinking Pattern Generation
The blinking pattern can be generated either by using a pre-recorded image/video or by the same tool via a
dedicated command-line option. If enabled, the class BlinkingPatternGenerator
is started.
SpatioTemporalContrast Filter
The filter Metavision::SpatioTemporalContrastAlgorithmT
is used to filter the noise and reduce
the number of events to process.
Blinking Frame Generator Algorithm
The Metavision::BlinkingFrameGeneratorAlgorithm
detects events triggered by the blinking
pattern. The implementation is straightforward as it considers a pixel has blinked when at least two events with
different polarities have been triggered at that location within a given time window.
The algorithm is asynchronous in the sense that it produces a binary frame of blinking pixels every time enough blinking pixels have been detected. In other words, for each input buffer of events, this algorithm might produce 0, 1, or N binary frames.
Discrete Fourier Transform Class
The DftHighFreqScorer
class uses the Metavision::DftHighFreqScorerAlgorithm
to compute the Discrete Fourier Transform (DFT)
on the input binary frame. The DFT is then used to compute a focus score.
The class DftHighFreqScorer
produces a frame for visualization which contains the DFT score.
The Metavision::DftHighFreqScorerAlgorithm
is synchronous as it produces a new DFT score for each input
frame. To reduce the computation cost, the algorithm can be configured to check whether the input frame has changed
since the previous one. A new DFT score is then only produced in this case.
Period Frame Generation Algorithm
The Metavision::PeriodicFrameGenerationAlgorithm
is used to generate a frame from the events. The events are directly drawn
in the frame upon reception, and the frame is produced at a fixed frequency in the camera’s clock.
Frame Composer
The Metavision::FrameComposer
class creates a frame made of:
the raw events frame on the left
the blinking events frame on the right
the DFT score frame on the top right
The composed frame is produced at a fixed frequency in the camera’s clock in contrast to the input frames that might arrive at different and variable frequencies. Variable frequencies are due to asynchronous algorithms that might produce 0, 1, or N output(s) for each input.
Display
The frame produced by the Metavision::FrameComposer
is displayed using Metavision::Window
:
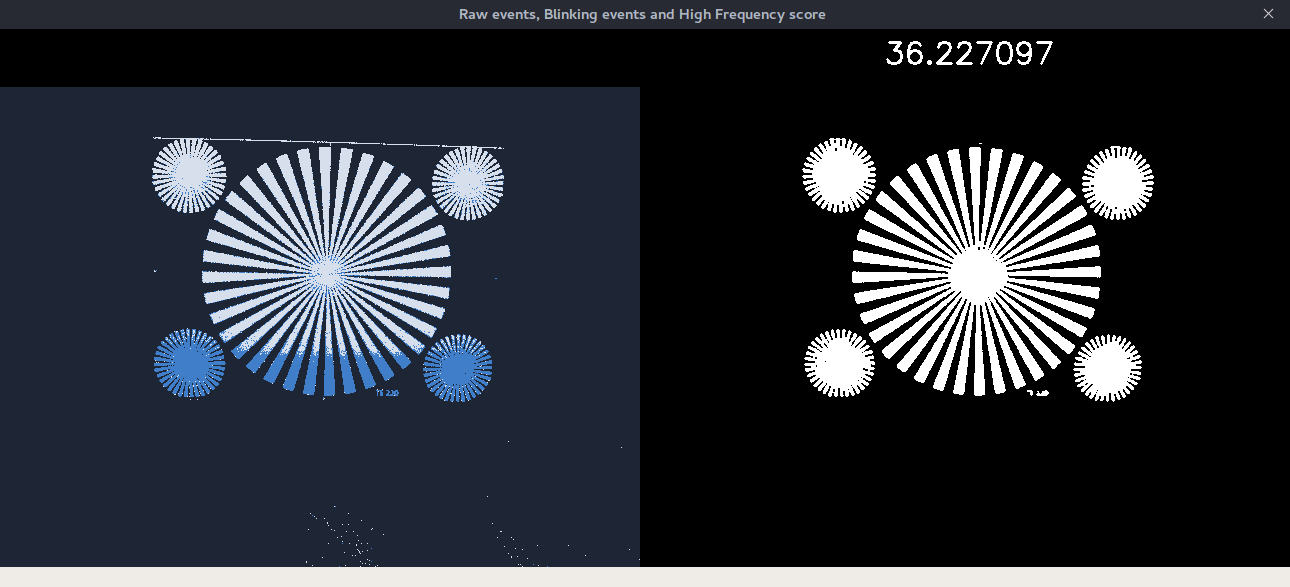
The printed score corresponds to the percentage of high frequencies (i.e. sharp details) in the image, so the higher the score the better.
When the option is enabled, the blinking pattern is also displayed in another window:
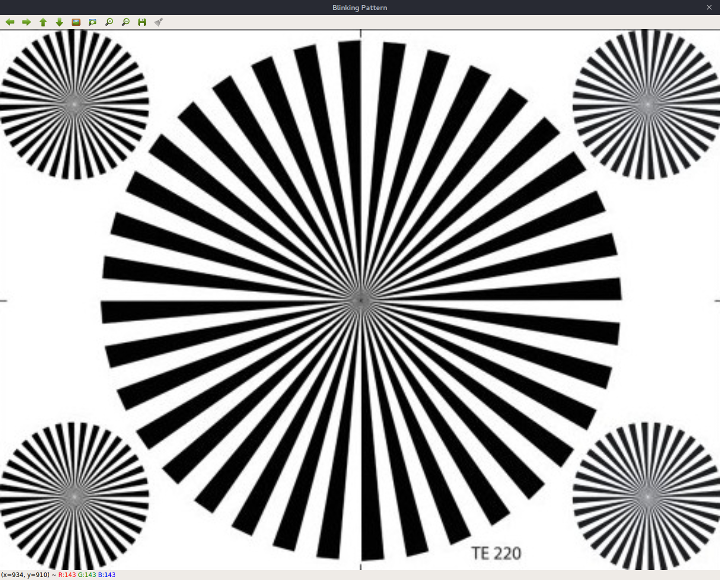