HDF5 Event File Format
Table of Contents
Definition
Metavision SDK allows to store event-based data in HDF5, a standard hierarchical self-describing file format using a lossless compression codec called ECF (Event Compression Format).
If you want to access the data of our HDF5 event files, you must use our ECF codec. It is delivered with Metavision SDK on both Ubuntu and Windows. It is also available as a shared library and an ECF filter plugin for HDF5 (with self-assigned filter code 0x8ECF) in the hdf5_ecf GitHub repository.
Warning
HDF5 event files should not be confused with HDF5 tensor files that can be created with our generate_hdf5 Python script as explained in the tutorial Precomputing features as HDF5 tensor files.
HDF5 Event Files Usage
HDF5 event files can be read with our applications (e.g. Metavision Studio), our C++ samples (e.g. Metavision Viewer) and our Python samples (e.g. Metavision Simple Viewer).
In the C++ API of the SDK, HDF5 event files are handled in Camera class (Metavision::Camera
) in the Stream module along with
the RAW and DAT file format. Our HDF5 event files can also be directly read and written using Metavision::HDF5EventFileReader
and
Metavision::HDF5EventFileWriter
.
In the Python API of the SDK, HDF5 event files can be read by Core module EventsIterator
.
At the moment there is no class to write HDF5 event file in Python.
Note
You can download some HDF5 event files in our Sample Recordings page, or convert one of your RAW file into HDF5 event format using File to HDF5 tool.
Format of HDF5 event files
To read and write HDF5 event files using the SDK, you are not required to know the internal structure of the files as the SDK will handle it for you. But if you want to leverage third-party tools, as introduced in the next section, it is useful to have some information about the format.
Our HDF5 event files are made of:
some attributes giving some meta-data about the file similarly to the header of the RAW files:
format
camera_integrator_name
plugin_integrator_name
etc.
two groups that contain the event data itself:
CD
: CD events
EXT_TRIGGER
: External Trigger Events
Each of the two groups has two dataset members:
events
: a compound dataset made of multiple fields describing the event (encoded with our ECF codec as mentioned above). For example, for CD events, the fields are:
x
: X coordinate of the event
y
: Y coordinate of the event
p
: Polarity of the event
t
: timestamp (in us) of the event
indexes
: a compound dataset that contains index information to locate the events in theevents
dataset. The events are indexed every 2000us. For CD events, the fields are:
id
: index of the event in theevent
dataset
ts
: timestamp of the event of indexid
But note that there is also an attribute called
offset
on theindexes
dataset that contains the value that is added to the timestamp of the event before storing its value in theindexes
.For example, if we have an HDF5 file with
offset
of -6384 andindexes
starting with those elements:
(0, -1)
(0, 0)
(77012, 2000)
(154954, 4000)
(234081, 6000)
Then it should be understood like this:
the event of
id
77012 has a timestamp of 8384 (2000+6384)the event of
id
154954 has a timestamp of 10384 (4000+6384)if you are looking for an event with timestamp ts=11000, then you have to add the offset (-6384) to obtain the timestamp value you should find in the
indexes
. So in that case you get 4616, hence the event with a timestamp close to 11000 will be found between the events ofid
154954 and 234081.Note
This
offset
is only used to optimize the storage of the HDF5 indexes. It should not be confused with the “shift” applied by the SDK when reading RAW files (see Timestamp Shifting page).
Using HDF5 events files with third-party tool
The HDF5 tools and API can be directly used on our HDF5 event files. The bindings of HDF5 in other languages such as h5py can also be used to manipulate the HDF5 event files produced by Metavision SDK.
HDF5 must be made aware of the ECF codec to correctly interpret the data contained in the files. When installing the SDK on Ubuntu or Windows, the codec and the plugin are installed. On Ubuntu, the HDF5 related packages can also be installed independently from the SDK:
sudo apt install hdf5-ecf-codec-dev hdf5-ecf-codec-lib hdf5-plugin-ecf hdf5-plugin-ecf-dev
Additionally, you need to set the HDF5_PLUGIN_PATH
environment variable to direct to the ECF codec plugin’s location.
Refer to the relevant installation guide to learn how to adjust this variable.
Note
Using custom HDF5 filter plugins is slower than using directly the Metavision SDK. This should be reserved for quick prototyping or applications where the performance is not important.
Example using hdfview
Here, we use hdfview to show the list of attributes of our driving sample HDF5 event file:
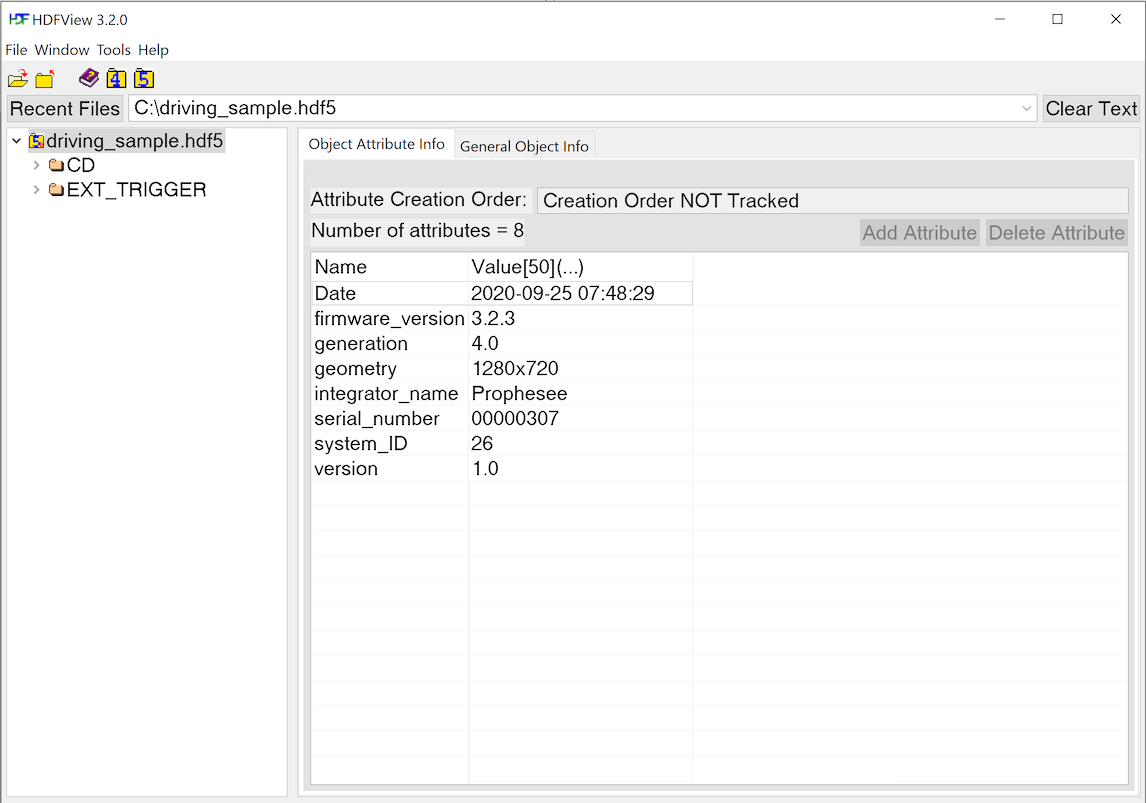
And here, we look at the structure (x, y, p, t) of the events
member of the CD
dataset:
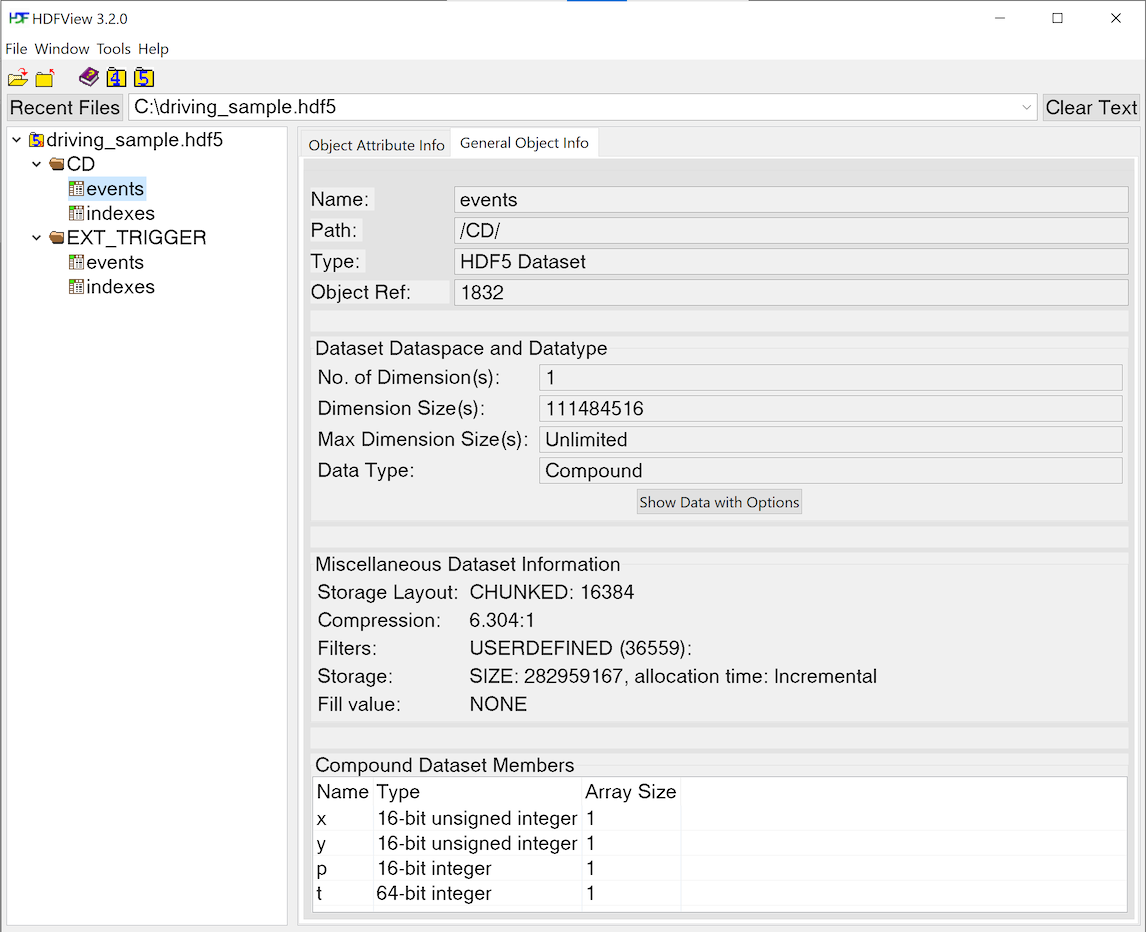
Example using h5dump
You can quickly extract information from one of our recording using h5dump.
For example, on Ubuntu, we can extract the resolution (via the geometry
attribute) of the turning spinner sample recording:
h5dump -a geometry spinner.hdf5
which would give the following output :
HDF5 "spinner.hdf5" {
ATTRIBUTE "geometry" {
DATATYPE H5T_STRING {
STRSIZE H5T_VARIABLE;
STRPAD H5T_STR_NULLTERM;
CSET H5T_CSET_ASCII;
CTYPE H5T_C_S1;
}
DATASPACE SCALAR
DATA {
(0): "640x480"
}
}
}
Or display the value of the first 3 events :
h5dump -d "/CD/events" -k 3 spinner.hdf5
which would output :
HDF5 "spinner.hdf5" {
DATASET "/CD/events" {
DATATYPE H5T_COMPOUND {
H5T_STD_U16LE "x";
H5T_STD_U16LE "y";
H5T_STD_I16LE "p";
H5T_STD_I64LE "t";
}
DATASPACE SIMPLE { ( 54165303 ) / ( H5S_UNLIMITED ) }
SUBSET {
START ( 0 );
STRIDE ( 1 );
COUNT ( 1 );
BLOCK ( 3 );
DATA {
(0): {
237,
121,
1,
0
},
(1): {
246,
121,
1,
0
},
(2): {
248,
132,
1,
0
}
}
}
}
}
Note
To use HDF5 tools
on Ubuntu, you may need to install an additional package :
sudo apt -y install hdf5-tools
Example using h5py
As a toy example, we want to count how many events occurred between two timestamps in a specified ROI :
import h5py
with h5py.File("spinner.hdf5", "r") as f:
evts = f["CD"]["events"]
print(len(evts[(evts['t']<49330) & (evts['t']>48270) & (evts['x']<300) & (evts['y']>300)]))
which would output :
33
Note
This is a simple and quite inefficient way of extracting information from an HDF5 recording. For better approaches, read the h5py documentation or use the Metavision SDK.