Note
This C++ sample has a corresponding Python sample.
Camera Stream Slicer using C++
The Camera Stream Slicer sample demonstrates how to utilize the CameraStreamSlicer
class from the Metavision SDK to divide and process event streams generated by a Camera
instance. This can be done based on either a fixed number of events or a specified time duration.
Expected Output
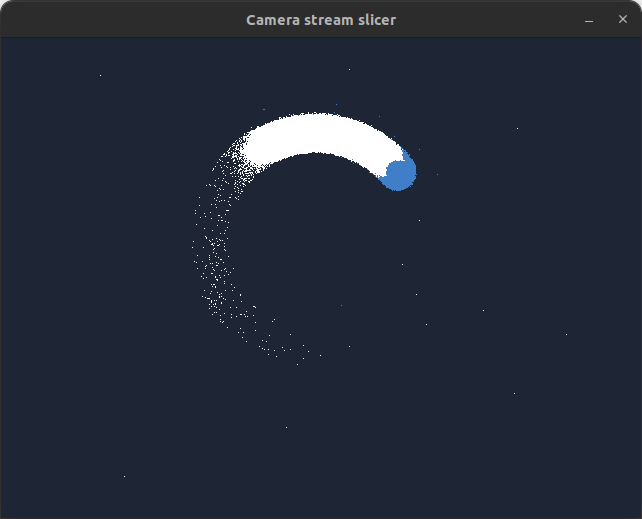
How to start
You can directly execute pre-compiled binary installed with Metavision SDK or compile the source code as described in this tutorial.
To start the sample based on recorded data, provide the full path to a RAW file (here, we use a file from our Sample Recordings):
Linux
./metavision_camera_stream_slicer -i spinner.raw
Windows
metavision_camera_stream_slicer.exe -i spinner.raw
To start the sample based on the live stream from your camera, run:
Linux
./metavision_camera_stream_slicer
Windows
metavision_camera_stream_slicer.exe
To check for additional options:
Linux
./metavision_camera_stream_slicer -h
Windows
metavision_camera_stream_slicer.exe -h
Code Overview
First we start by initializing a Camera
instance based on the parsed command line
arguments:
Metavision::Camera camera;
if (!config->record_path.empty()) {
camera = Metavision::Camera::from_file(config->record_path);
} else if (!config->serial_number.empty()) {
camera = Metavision::Camera::from_serial(config->serial_number);
} else {
camera = Metavision::Camera::from_first_available();
}
Next, we create a CameraStreamSlicer
instance by transferring the
ownership of the camera instance to it and setting the desired slicing mode:
Metavision::CameraStreamSlicer slicer(std::move(camera), config->slicing_condition);
Finally, we start slicing the event stream by iterating over the slicer. The information about the sliced events is printed to the console and the sliced events are displayed in a window:
for (const auto &slice : slicer) {
MV_LOG_INFO() << "ts:" << slice.t << "new slice of" << slice.n_events << "events";
frame_8uc3.create(height, width, CV_8UC3);
Metavision::BaseFrameGenerationAlgorithm::generate_frame_from_events(slice.events->cbegin(),
slice.events->cend(), frame_8uc3);
for (const auto &t : *slice.triggers) {
MV_LOG_INFO() << "ts:" << t.t << "new external trigger with polarity" << t.p;
}
cv::imshow("Camera stream slicer", frame_8uc3);
const auto cmd = cv::waitKey(1);
if (cmd == 'q')
break;
}