Note
This Python sample has a corresponding C++ sample.
Camera Stream Slicer using Python
The Camera Stream Slicer sample demonstrates how to utilize the CameraStreamSlicer
class from the Metavision SDK to divide and process event streams generated by a Camera
instance. This can be done based on either a fixed number of events or a specified time duration.
Expected Output
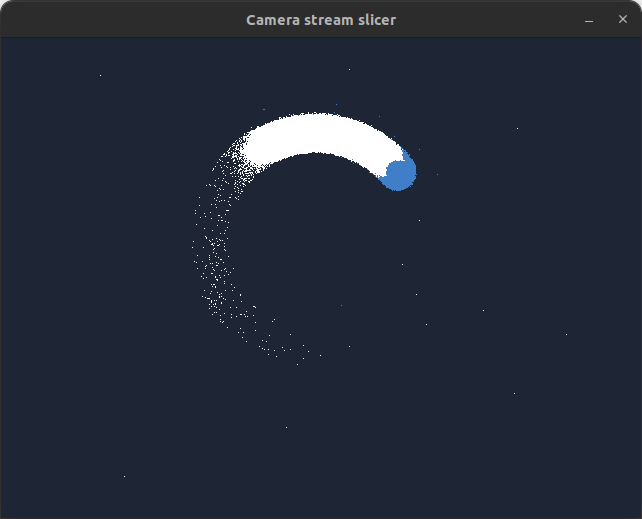
How to start
To start the sample based on recorded data, provide the full path to a RAW file (here, we use a file from our Sample Recordings):
python metavision_camera_stream_slicer.py -i spinner.raw
To start the sample based on the live stream from your camera, run:
python metavision_camera_stream_slicer.py
To check for additional options:
python metavision_camera_stream_slicer.py -h
Code Overview
First we start by initializing a Camera
instance based on the parsed command
line arguments:
if args.camera_serial_number:
camera = Camera.from_serial(args.camera_serial_number)
elif args.input_event_file:
hints = FileConfigHints()
hints.real_time_playback(args.real_time_playback)
camera = Camera.from_file(args.input_event_file, hints)
else:
camera = Camera.from_first_available()
Next, we create a CameraStreamSlicer
instance by transferring the
ownership of the camera instance to it and setting the desired slicing mode:
slicer = CameraStreamSlicer(camera.move(), args.slice_condition)
Note
The camera instance is transferred to the slicer in a similar way it would be done in C++ by using the move semantic. It means that any variable holding the moved camera instance after this point will be invalid and should not be used anymore (i.e. undefined behavior otherwise). Under the hood, the move() function will return a special object that will hold the ownership of the camera instance. This object can only be used to instantiate a slicer instance. An exception will be thrown if the user tries to use this special object more than once.
Finally, we start slicing the event stream by iterating over the slicer. The information about the sliced events is printed to the console and the sliced events are displayed in a window:
for slice in slicer:
EventLoop.poll_and_dispatch()
print(f"ts: {slice.t}, new slice of {slice.events.size} events")
BaseFrameGenerationAlgorithm.generate_frame(slice.events, frame)
window.show_async(frame)
if window.should_close():
break